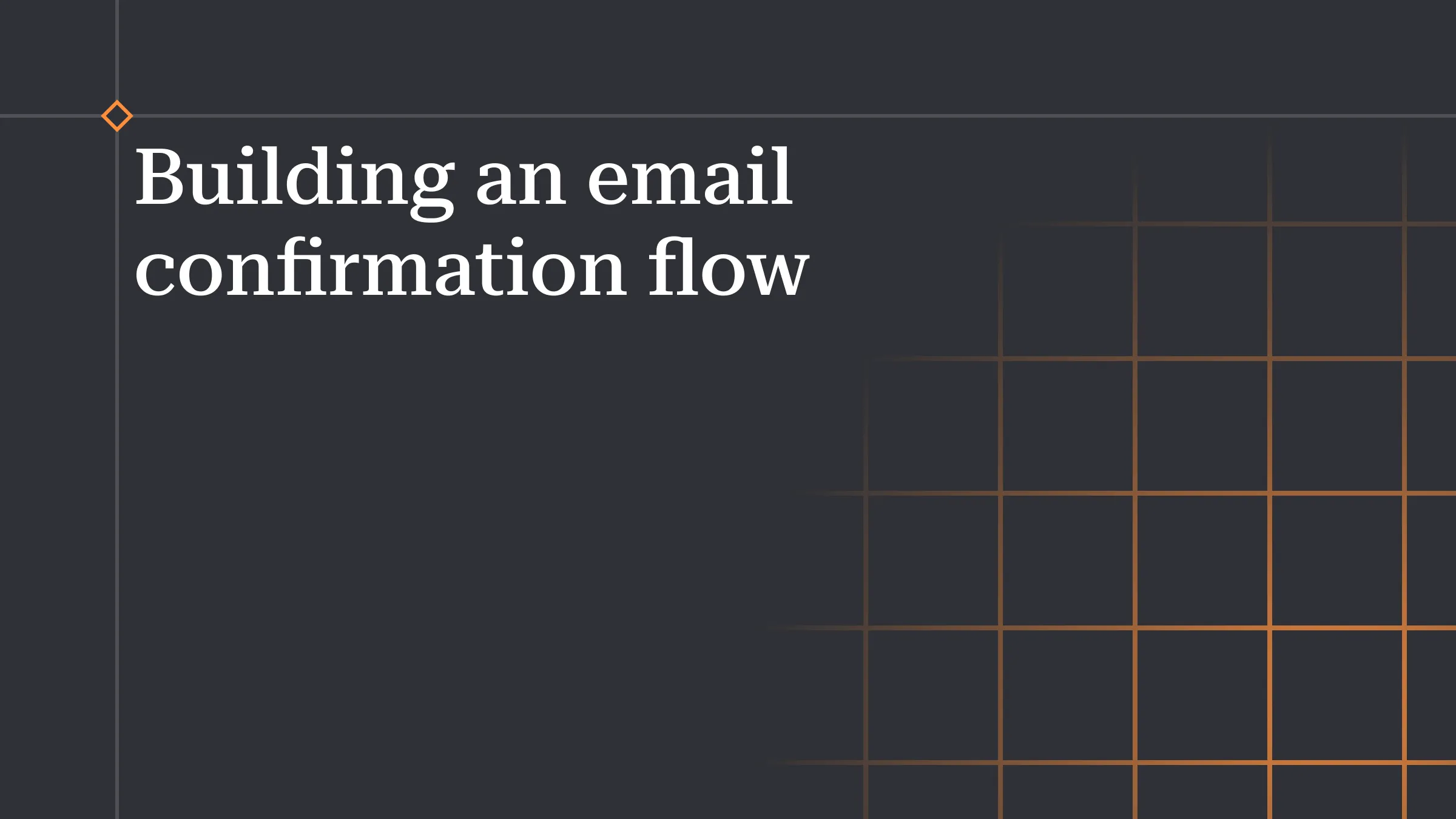
Building an Email Confirmation Flow with Sailhouse, Netlify, and Resend
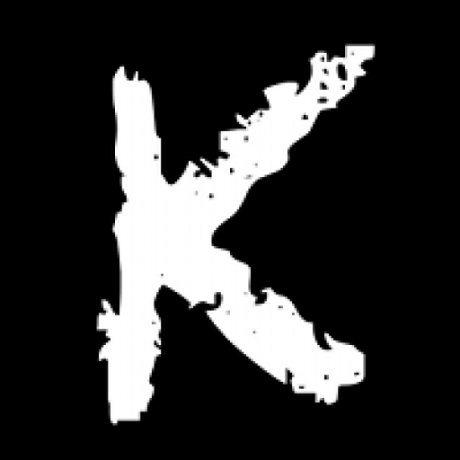
TL;DR: I built an open-source email confirmation system using Sailhouse’s event architecture and Netlify serverless functions, using Resend for the emails. Grab it, hack it, use it! github.com/sailhouse/email-confirmation
1. Introduction: Why Email Confirmation Matters
It’s one of those things every project needs, but nobody’s particularly excited to implement. These flows do important stuff:
- They stop the bots (mostly)
- Keep you on the right side of privacy laws
- Show users you’re not going to spam them into oblivion
- Help maintain a list of people who actually want your emails
After 20 years of working with these things (and seeing them break in production 😅), I wanted something simple that just works without becoming another headache.
2. Common Email Confirmation Considerations
Let’s be real about what we all think when approaching this problem:
- “Do I really need to build this again?” - The eternal build vs. buy dilemma
- “This shouldn’t be so complicated” - Yet somehow, it always is
- “I don’t want to pay $X per month just for confirmations” - Especially for side projects
- “I don’t need a full on CRM/growth hacking platform” - A lighter solution for informational messages can be enough
- “But I need it to work with my weird edge case” - Because requirements are never standard
- “I want control without the maintenance nightmare” - The developer’s dream
I finally decided to build something reusable. Classic developer move, right? “I’ll save time in the long run!” 🙃 But I did enjoy myself doing it. So there’s that.
3. The Power of Event-Driven Architecture with Sailhouse
Event-driven architecture is one of those approaches that just makes sense once you try it:
- Components talk through events, not direct calls - way less “you changed your API and broke my stuff” moments
- If one part breaks, the whole system doesn’t necessarily collapse
- Handles traffic spikes gracefully - events just queue up
- It can even makes the system easier to reason about - “this happens, then that happens”
We built Sailhouse to make this kind of architecture accessible without needing a PhD in distributed systems. It’s the infrastructure we both wish we’d had for the last decade of projects.
4. Solution Architecture Overview 🏗️
Here’s what’s going on under the hood:
- Sailhouse: Handles all the event passing and sequencing
- Netlify Functions: Lightweight serverless bits that process the events
- Events for state: No database needed - the event stream itself maintains state
- Pluggable email delivery: Works with whatever you’re using to send emails. In production we are using Resend.
Since we are using this to confirm subscription via email to our changlog The flow is pretty straightforward:
- User submits their email
- We fire off a
changelog-subscription
event (steps 1 and 2 are from our website) - Sailhouse processes the event, and there is a subscription setup in Sailhouse to ping a Netlify Function to process the event and create a secure token
- Email with confirmation link gets sent
- User clicks the link (hopefully!)
- System verifies the token and publishes
changelog-confirmation
, and saves the email address - The confirmed email can then be handled either via another function, or subscribing to events or, indeed be used to update Slack.
No SQL queries, no complex state management. Events all the way down!
Oh, I should mention - we’re using Netlify Blob Store to persist subscriber data. It’s a nice little feature that Netlify provides out of the box, so we don’t need to set up a separate database. The events drive the system, and the blob store gives us somewhere to stash the data for later retrieval or processing. It’s surprisingly powerful for something that requires zero setup!
5. Implementation Highlights
I’ve focused on making this both solid and developer-friendly:
- Everything communicates through well-defined events
- Tokens are properly secured (because security matters)
- Email templates you can actually customise without fighting the system; thank you react-email
- Sensible defaults but configurable where it counts
- Error handling that won’t leave you debugging at 2am
The system starts with the subscription-handler.ts
function which receives events from Sailhouse and validates they were truly from Sailhouse
const shSignature = req.headers.get('Sailhouse-Signature');
const body = await req.text();
if (!shSignature) {
return new Response('Missing Sailhouse signature', { status: 401 });
}
const valid = sailhouseClient.verifyPushSubscription(shSignature, body, SIGNATURE);
if (!valid) {
return new Response('Invalid Sailhouse signature', { status: 401 });
}
The service uses React Email to create responsive, customisable email templates:
// In ConfirmationEmail.tsx
export const ConfirmationEmail: React.FC<ConfirmationEmailProps> = ({
confirmationUrl, // Generated for you with an encoded token
mailingListName, // Name of the list - we used Changlog here
companyName, // Name to be used in the email subject line - we use Sailhouse here
baseUrl, // url of your site
}) => {
// Email template with your brand's styling
}
The email utility supports multiple providers with a simple abstraction:
// In email.ts
export const sendConfirmationEmail = async (options: SendEmailOptions): Promise<boolean> => {
const provider = getEmailProvider();
switch (provider) {
case 'postmark':
return sendWithPostmark(options);
case 'resend':
return sendWithResend(options);
default:
console.error('No email provider configured');
return false;
}
};
When users click the confirmation link, the confirm.ts
function handles the process and if it can decode the email, it publishes a confirmation event to Sailhouse
await sailhouse.publish(process.env.CONFIRMATION_TOPIC || 'changelog-confirmation', {
email,
confirmed: true,
timestamp: new Date().toISOString(),
});
Security is super important with confirmation flows. I’ve implemented HMAC-based tokens to make sure nobody can tamper with our confirmation links.
// Create HMAC signature
const hmac = crypto.createHmac('sha256', process.env.TOKEN_SECRET);
hmac.update(email);
const signature = hmac.digest('hex');
// Combine email and signature in the token
const tokenData = {
email,
signature,
};
This approach combines the email address with a cryptographic signature, so we can verify it hasn’t been tampered with when the user clicks the link. And since we’re developers who appreciate good tools, I even included a utility script for testing token generation and verification during development.
For storing confirmed subscribers, I’m leveraging Netlify Blob Store which gives us persistence without any database setup:
await blobStore.setJSON(email, {
email,
timestamp,
});
The code is designed to be easily customisable:
- Environment variables control email providers, sender information, and topics
- HTML and React templates can be modified to match your company’s style for both the web pages and the email that is sent
- Sailhouse topics can be configured to fit your event schema
6. Developer-Friendly Customisation Points
Here’s where it gets fun - this thing is designed to be hacked on:
- Super quick setup: Clone the repo, connect to Netlify, setup a subscription from Sailhouse pointing to your site on Netlify, and you’re literally done in under 5 minutes. Not even exaggerating.
- Simple config: Just copy
.env.example
and drop in your values. You can even change the event topic name. - Email templates: They’re just React with some basic props passed in. No fancy template engine to learn. Want to add your logo and brand colors? Just do it!
- Add your own logic: The subscriber pattern makes it dead simple to extend once a confirmation has happened
I’ve added analytics tracking, CRM updates, and even a special welcome sequence for certain domains - all without touching the core code. That’s the beauty of event-driven design.
To demonstrate this, I also built in a summary reporting feature that sends updates to Slack. This is super handy for keeping track of new subscribers without having to log into another dashboard. The cool thing is this all happens automatically using a Sailhouse Cron job. You just set up a recurring event in Sailhouse that triggers this function to run daily, and boom - automated reporting! And want to switch email providers? Easy. The system supports both Postmark and Resend out of the box, and adding another is just a matter of implementing a single function that follows the pattern. Just change an environment variable to switch providers - no code changes needed. Oh, and if you’re wondering how the Netlify integration works - it just does! The service automatically uses Netlify’s context object to determine your site URL, so there’s no configuration needed. One less thing to worry about.
7. Beyond Email Confirmation: Other Cool Stuff You Could Build
Once you’ve got this pattern down, you can apply it to tons of other flows:
- Two-factor auth flows: “Enter the code we just sent you”
- Preference management: “Click here to update your settings”
- Approval chains: “Bob needs to approve this before it proceeds”
- Notification systems: “Something happened that you care about”
The nicest part is how little code you need to adapt for these use cases. The event-driven pattern scales beautifully to different problems.
8. Why We Built This At Sailhouse
Totally honest moment - we built this for a few reasons:
- Dogfooding our own product: Nothing tests a platform like actually using it. Yes I broke it. This is mandatory doing such things 🤣
- Scratching our own itch: We needed this for our projects anyway
- Sharing something useful: Why not be helpful to others out there?
- Showing off event-driven patterns: It’s surprisingly elegant for these flows
- Learning from real usage: Building real things teaches you what matters
This wasn’t some grand plan to create the ultimate email confirmation solution - it was just us solving a common problem in a way that made sense, then thinking “hey, others might find this useful too.”
9. Getting Started
Want to try it out? It’s super simple:
- Fork the repo: github.com/sailhouse/email-confirmation
- Set your environment variables
- Hit the Netlify deploy button (or hook it up to your preferred serverless platform)
- Setup the push subscription on Sailhouse
- For bonus points, set up a Sailhouse Cron job to trigger daily summary reports:
- Create a cron trigger in Sailhouse (e.g., daily at 9 AM)
- Have it publish to a topic that has a push subscription to your
/api/summary
endpoint - Configure the
CONFIRMATION_SUBSCRIPTION
environment variable to point to your subscription
- Start using the events in your app
Found a bug? Have an idea for improvement? PRs are absolutely welcome! This is a community tool, so help make it better.
10. Wrapping Up
Email confirmation isn’t the most exciting part of your app, but it’s one of those critical pieces of infrastructure that needs to be rock solid. This solution gives you a lightweight, scalable approach that you can set up in minutes and customise to your heart’s content.
The event-driven architecture makes it remarkably easy to extend, debug, and reason about - which means less time fighting with infrastructure and more time building the stuff that makes your product unique.
Give it a spin, hack it to pieces, make it your own! And definitely let me know what you build with it - I love seeing how people extend and improve these patterns.
Happy coding!